.fadeOut()
要素をフェードアウトで非表示にします。
- .fadeOut( [duration] [, callback] ) 1.0追加
- .fadeOut( [options] ) 1.0追加
- .fadeOut( [duration] [, easing] [, callback] ) 1.4.3追加
- 解説
- デモ
.fadeOut( [duration] [, callback] ) 1.0追加
戻り値:jQuery
引数 | 説明 |
---|---|
[duration] | フェードするアニメーション時間を指定します。 |
[callback] | アニメーション完了後に実行したい関数を指定します。 |
.fadeOut( [options] ) 1.0追加
戻り値:jQuery
引数 | 説明 |
---|---|
options |
型: メソッドに渡すオプションのマップになります。 |
.fadeOut( [duration] [, easing] [, callback] ) 1.4.3追加
戻り値:jQuery
引数 | 説明 |
---|---|
[duration] | フェードするアニメーション時間を指定します。 |
[easing] | アニメーションの変化の種類を指定します。 |
[callback] | アニメーション完了後に実行したい関数を指定します。 |
解説
.fadeOut()
メソッドは、一致した要素のopacity(不透明度)をアニメーションします。
opacityが0に達すると、display
のstyleがnone
に設定されるため、
その要素がページのレイアウトに影響を与えなくなります。
durationへの数値はミリ秒として扱われ、高い値はアニメーションを遅くし、低い値はアニメーションを速めます。
文字列の'fast'
と'slow'
は、
それぞれ200
と600
ミリ秒のdurationとして扱われます。
他の文字列が指定された場合、またはdurationパラメーターが省略された場合、
デフォルトの400
ミリ秒が使用されます。
下記のような単純な画像の要素をアニメーションさせることも可能です。
<div id="clickme">
Click here
</div>
<img id="book" src="book.png" alt="" width="100" height="123">
初めに表示されていた要素を、ゆっくり非表示にします。
$( "#clickme" ).click(function() {
$( "#book" ).fadeOut( "slow", function() {
// アニメーション完了時に実行させたい処理
});
});
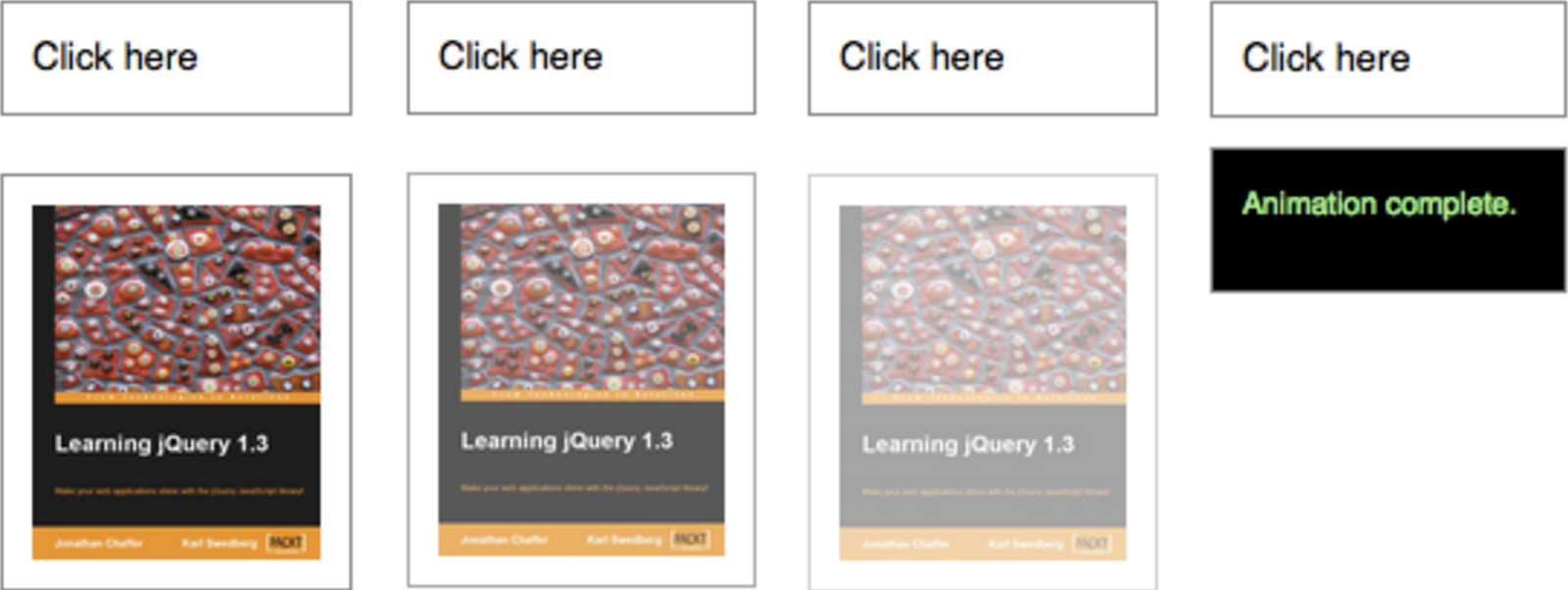
注意:
不要なDOM操作を避けるために、.fadeOut()
は既に隠されようとしている要素を、隠すことをしません。
jQueryが要素を隠そうとしているか否かは、
:hiddenセレクタで確認します。
イージング(easing)
jQuery 1.4.3からは、イージング(easing)関数の文字列を任意に指定できるようになりました。 イージング関数は、アニメーションの異なるポイントでの進行の速度を指定するものです。
jQueryライブラリで指定できるイージングは、デフォルトのswing
と、
一定のペースで進行するlinear
のみです。
より多くのイージング関数を使用するにはプラグインが必要で、
特に有名なのはjQuery UIになります。
コールバック関数
指定された場合、アニメーションが完了した際に実行されます。
これは、続けて異なるアニメーションを実行したい際に便利です。
このコールバックは引数を送りませんが、this
にはアニメーションされているDOM要素が設定されています。
もし、複数の要素がアニメーションされている場合、
コールバックは一致した要素ごとに実行されるのであり、アニメーションごとに実行されるわけでは無いことに注意しなければいけません。
jQuery 1.6からは.promise()メソッドを結合して、 deferred.done()メソッドで全ての一致している要素のアニメーションの完了時に、 単一のコールバックを実行することが可能になりました。(.promise()の例を参照)
アニメーションのOFF
-
.fadeIn()
を含む全てのjQueryのエフェクトは、jQuery.fx.off = true
にすることで実際にはdurationを0に設定することで、 グローバル全体でOFFにすることが可能です。 詳細は、jQuery.fx.offを参照してください。
デモ
全てのP要素がフェードアウトし、600ミリ秒でアニメーションが完了します。
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>fadeOut demo</title>
<style>
p {
font-size: 150%;
cursor: pointer;
}
</style>
<script src="https://code.jquery.com/jquery-1.10.2.js"></script>
</head>
<body>
<p>
If you click on this paragraph
you'll see it just fade away.
</p>
<script>
$( "p" ).click(function() {
$( "p" ).fadeOut( "slow" );
});
</script>
</body>
</html>
クリックしたSPAN要素部分がフェードアウトします。
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>fadeOut demo</title>
<style>
span {
cursor: pointer;
}
span.hilite {
background: yellow;
}
div {
display: inline;
color: red;
}
</style>
<script src="https://code.jquery.com/jquery-1.10.2.js"></script>
</head>
<body>
<h3>Find the modifiers - <div></div></h3>
<p>
If you <span>really</span> want to go outside
<span>in the cold</span> then make sure to wear
your <span>warm</span> jacket given to you by
your <span>favorite</span> teacher.
</p>
<script>
$( "span" ).click(function() {
$( this ).fadeOut( 1000, function() {
$( "div" ).text( "'" + $( this ).text() + "' has faded!" );
$( this ).remove();
});
});
$( "span" ).hover(function() {
$( this ).addClass( "hilite" );
}, function() {
$( this ).removeClass( "hilite" );
});
</script>
</body>
</html>
2つのDIV要素がフェードアウトします。 1つは"linear"イージングで、もう1つはデフォルトである"swing"イージングが使用されています。
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>fadeOut demo</title>
<style>
.box,
button {
float: left;
margin: 5px 10px 5px 0;
}
.box {
height: 80px;
width: 80px;
background: #090;
}
#log {
clear: left;
}
</style>
<script src="https://code.jquery.com/jquery-1.10.2.js"></script>
</head>
<body>
<button id="btn1">fade out</button>
<button id="btn2">show</button>
<div id="log"></div>
<div id="box1" class="box">linear</div>
<div id="box2" class="box">swing</div>
<script>
$( "#btn1" ).click(function() {
function complete() {
$( "<div>" ).text( this.id ).appendTo( "#log" );
}
$( "#box1" ).fadeOut( 1600, "linear", complete );
$( "#box2" ).fadeOut( 1600, complete );
});
$( "#btn2" ).click(function() {
$( "div" ).show();
$( "#log" ).empty();
});
</script>
</body>
</html>
© 2010 - 2017 STUDIO KINGDOM